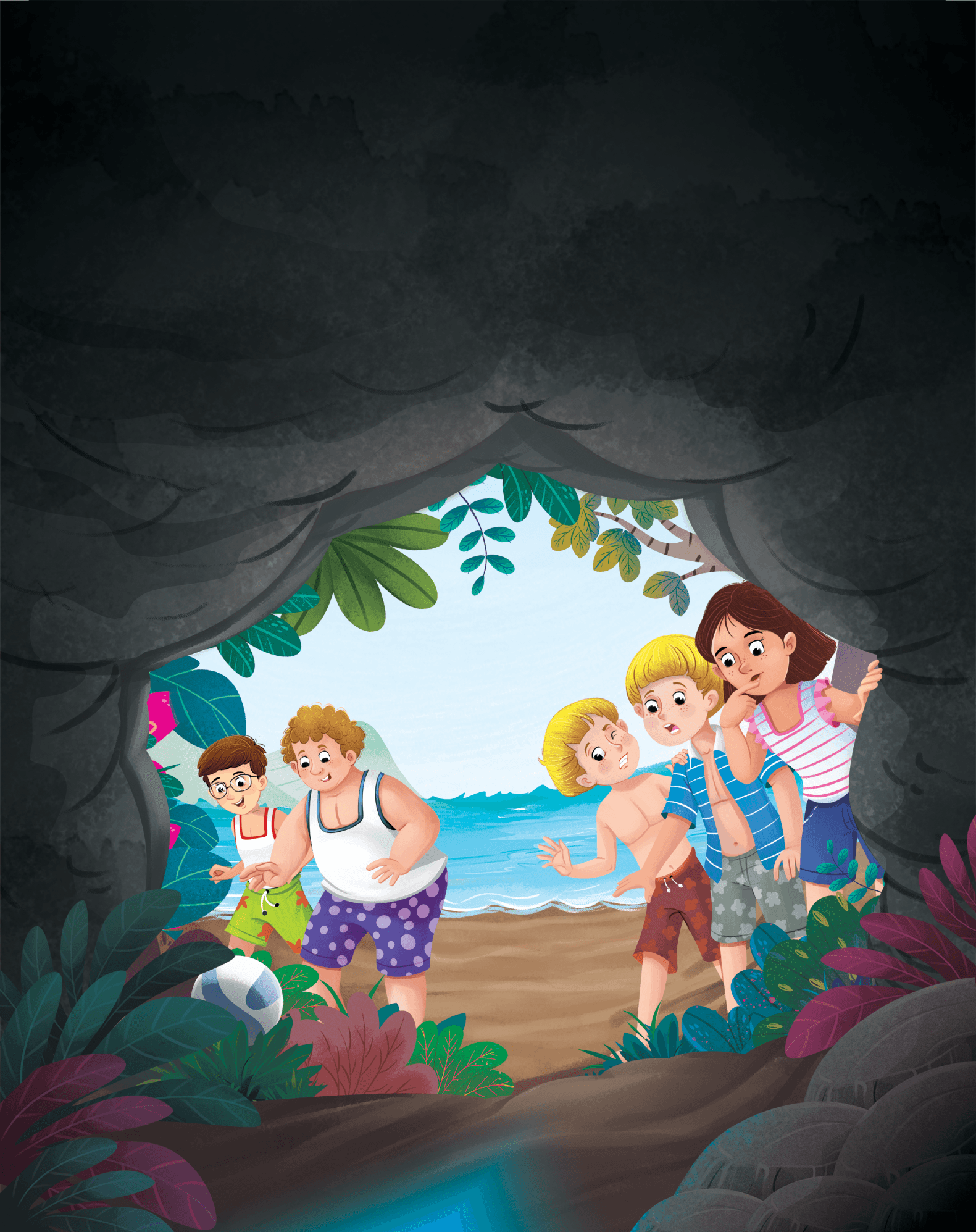
Beginners Guide to Robotics
Robo Explorers 1
Buzzer
What is Buzzer
A buzzer is a small device that makes a sound when electricity flows through it. Think of it like a tiny musical instrument!
How It Works
Electricity Makes It Move:
When you send electricity into the buzzer, it makes a small metal part inside it move or vibrate very quickly.Vibrations Create Sound:
These fast movements push against the air around the buzzer, creating waves in the air. These waves are called sound waves.You Hear the Buzz:
Your ears pick up these sound waves as a buzzing sound, just like a beep!
How to turn on buzzer on Yellow Tyro Board
Code
Checking the Button:
The code asks, "Is the buzzer button pressed?" When the button is pressed, it sends a special signal (LOW) to the Arduino.
Beeping the Buzzer:
If the button is pressed, the code turns the buzzer on. This makes it beep.Short Beep:
The buzzer stays on for a very short time (100 milliseconds), so you hear a quick beep.Turning Off:
After 100 milliseconds, the code turns the buzzer off, so the beep stops.Waiting a Little:
Then the code waits 200 milliseconds. This pause makes sure the Arduino doesn't think you pressed the button many times quickly (this is called debouncing).
Fun Activity!
Now that you understand how the buzzer works and have seen the main code pre-programmed in the Yellow Tyro board, let's have some fun! Below is a sample Arduino code that plays a cheerful "Jungle Bells" tune whenever you press the button. Simply upload this code to your board, press the button, and enjoy the musical surprise!
Explanation
Pin Setup:
The buzzer is connected to digital pin 8, and the button is connected to analog pin A1. We set the buzzer as an output and the button as an input. (This sketch assumes you’re using an external pull-down resistor so that the button reads LOW when not pressed and HIGH when pressed.)Button and Tune Logic:
In theloop()
, the code checks if the button reads HIGH and if the tune hasn't been played yet (using thetunePlayed
flag). When both conditions are true, theplayTune()
function is called to play the melody.
When the button is released (reads LOW), thetunePlayed
flag is reset to allow the tune to be played again on the next press.Playing the Tune:
TheplayTune()
function cycles through themelody
array, calculates how long each note should sound (using thenoteDurations
array), and uses thetone()
function to play each note on the buzzer. After each note, it pauses briefly before moving to the next note.
Thank you for exploring our buzzer page—a place where sound meets creativity! As you continue your Robo Explorer journey, may every project inspire you to think bigger and brighter. Now, you can proceed to the next chapter, where we dive into the fascinating world of potentiometers. Enjoy the adventure!