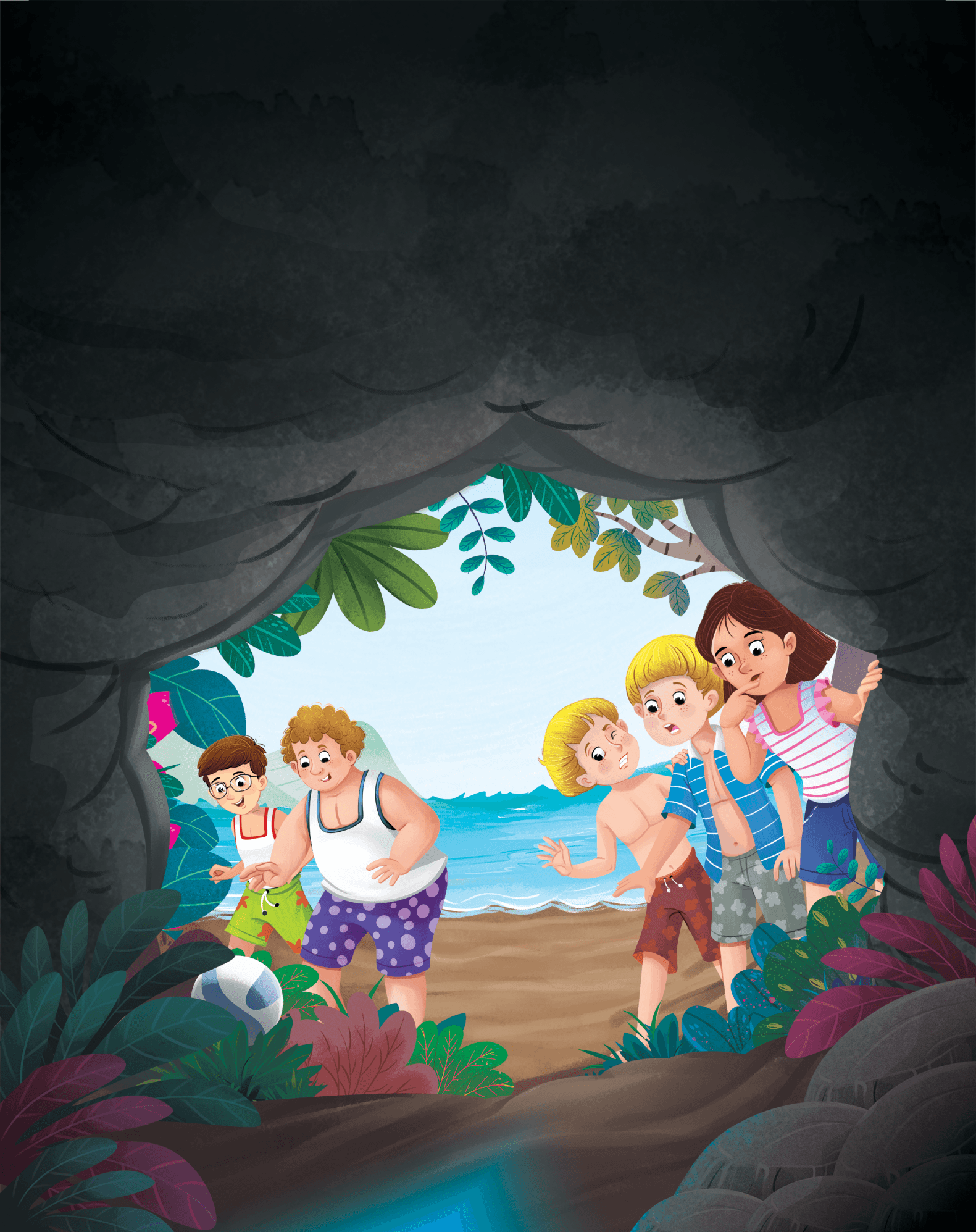
Beginners Guide to Robotics
Robo Explorers 1
Yellow LED
What is an LED?
Tiny Light:
An LED is like a mini flashlight that never burns out like a regular light bulb.Special Diode:
A diode is a tiny part that only lets electricity flow in one direction, which helps control when the light turns on.
The Physics Behind It
Imagine you’re at a playground:
Electrons Are Like Kids on a Slide:
Inside the LED, there are tiny particles called electrons. They are a bit like kids waiting at the top of a slide.Sliding Down (Releasing Energy:
When you send electricity into the LED, these electrons get excited and start sliding down a "slide" inside the LED.Light as a Spark:
As the electrons slide down (or drop from a high energy level to a lower one), they give off a little burst of energy. That burst of energy comes out as light—just like a little spark that makes the LED glow.
Turning on LED on Yellow Tyro Board
Anode and Cathode: The Two Sides of an LED
An LED has two tiny legs (or sides), and each side has a special name:
Anode:
This is the longer leg of the LED. It’s like the door where the electricity comes in. The anode is connected to the positive side (the +) of the battery.Cathode:
This is the shorter leg of the LED. It’s like the exit door for the electricity. The cathode is connected to the negative side (the -) of the battery.
For the LED to light up, the electricity must flow in one direction: from the battery's positive side, through the resistor, into the anode, out through the cathode, and back to the battery’s negative side.
Code Snippet Breakdown
Pin Setup:
In the setup()
function, we loop through the ledPins
array and configure each pin as an output using pinMode()
. This allows the Arduino to send signals to these pins to turn the LEDs on or off.
Turning LEDs On:
In theloop()
function, we loop through the same array and set each LED pin HIGH withdigitalWrite()
, which turns the LEDs on continuously.
This snippet is the part of the main code that takes care of lighting up the LEDs.
Fun Activity!
Get ready to take your Yellow Tyro Board to the next level with this creative and interactive activity! In this project, you’ll use your yellow LED to translate Morse code into light signals. By entering a series of dots (.) and dashes (-) through the Arduino Serial Monitor, you’ll make the LED blink in real-time, following the rhythm and structure of classic Morse code.
This activity is a fun way to learn how code, timing, and communication come together in electronics. You’ll explore how your program reads input from the user, processes each symbol, and then uses the yellow LED connected to pin D3 to blink out your custom message. Short blinks represent dots, longer ones represent dashes, and pauses separate letters—just like in real Morse communication.
How It Works
Setup and Initialization:
The LED is connected to pin D3 (defined as
LED_PIN
).The code starts Serial communication so that you can type in your Morse code using the Serial Monitor.
A message is printed to the Serial Monitor instructing you how to enter the Morse code.
Reading User Input:
In the
loop()
function, the code checks if any data has been entered through Serial.It reads the entire line (until a newline) as a Morse code string and trims extra spaces.
Processing the Morse Code:
The
processMorseCode()
function goes through each character of the string.If it finds a dot (
.
), the LED blinks for 250 ms.If it finds a dash (
-
), the LED blinks for 750 ms.If it finds a space (
A short gap is added between each dot or dash within the same letter.
Upload this sketch to your Yellow Tyro Board, open the Serial Monitor (set to 9600 baud), and type in your Morse code message (e.g., .- -...
) to see the LED blink in Morse code. Enjoy experimenting with your very own Morse code blinker!